I’ve made a little progress on this case and found some interesting things.
First, thanks again to @gaoxi for pointing out the radius/diameter mixup.
Comment: I think it would be best for this input to be optional. It can be computed from the semiaxes, and for non-particle_input.dat
cases (automatic particle generation), if the diameter is not specified it is computed from the semiaxes. So why make users specify it here?
Once I corrected the value in the file, I noticed something interesting. In the VTK window:
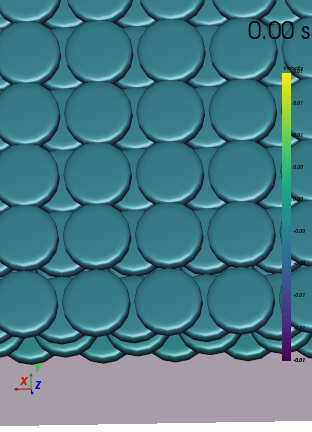
when we look closely they do seem to be overlapping a bit.
This is quite strange because yesterday when I looked at the particles they were not touching at all:
I think the only thing that has changed is the diamater specified in particle_input.dat
. But that should not affect how the particles are displayed in the VTK window, because, again, the superquadrics are completely described by the semiaxes and m,n exponents. Perhaps there is a bug in the visualization code, I will follow up on this.
So I decided to take seriously the thousands of
overlap between two superquadric particles is too large!
messages on the screen.
Looking at your data file, the minimum spacing between the particles in the y-direction is 0.0131989
which is very close to the bounding sphere diameter, it looks like it is close enough to cause trouble:
0.0131989 - 0.01278799737489740 = 0.000410
too close for comfort.
Here’s a little Python script that adds a little breathing room:
#!/usr/bin/env python
import numpy as np
input = open('particle_input.dat')
output = open('particle_input.dat.new', 'w')
# copy header
for lno in range(38):
output.write(input.readline())
# load numeric data
data = np.loadtxt(input)
# rescale
data *= [1, 1.1, 1]
# write output
for row in data:
row.tofile(output, sep=' ')
output.write('\n')
(Rename particle_input.dat.new
to particle_input.dat
after running this.)
After doing this the overlap is too large!
messages went away, at least for a while. I am still getting a crash, but it runs a little further before crashing, and goes a lot faster (I am also running 8-thread SMP which helps a lot).
Here’s the particles with a little more space:
and here’s a little movie showing how far we’ve come:
More to come …